Get full address from geo coordinates using Python for free.
Recently, I have been tasked to retrieve full addresses from geo coordinates for over 4k records. Nominatim is a great tool for this task, but it has a limit of 1 request per second.
You can read this on Medium here.
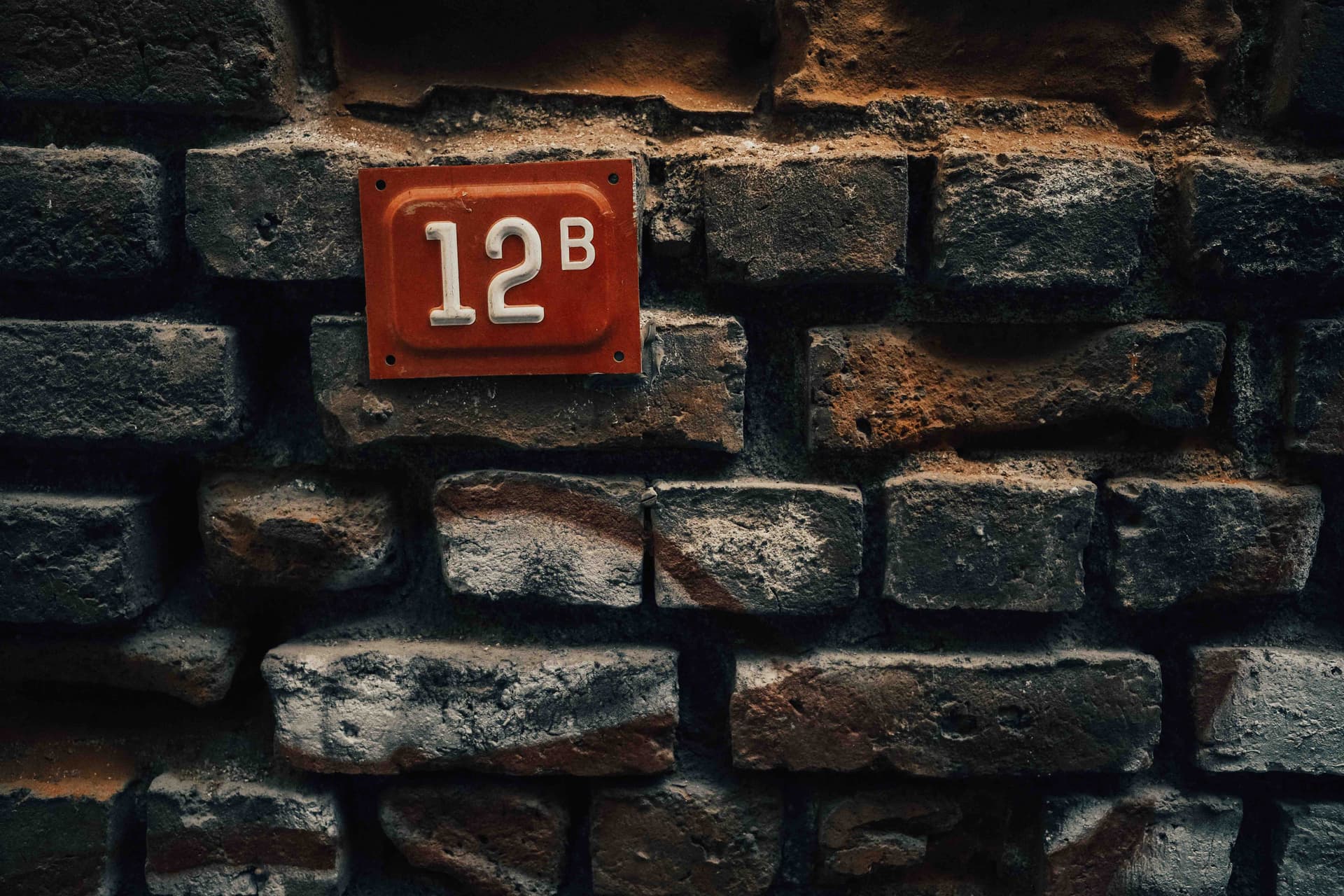
IMPORT LIBRARIES
import pandas as pd
from geopy.geocoders import Nominatim
from geopy.extra.rate_limiter import RateLimiter
IMPORT DATA
df = pd.read_csv("france_ria_locations.csv")
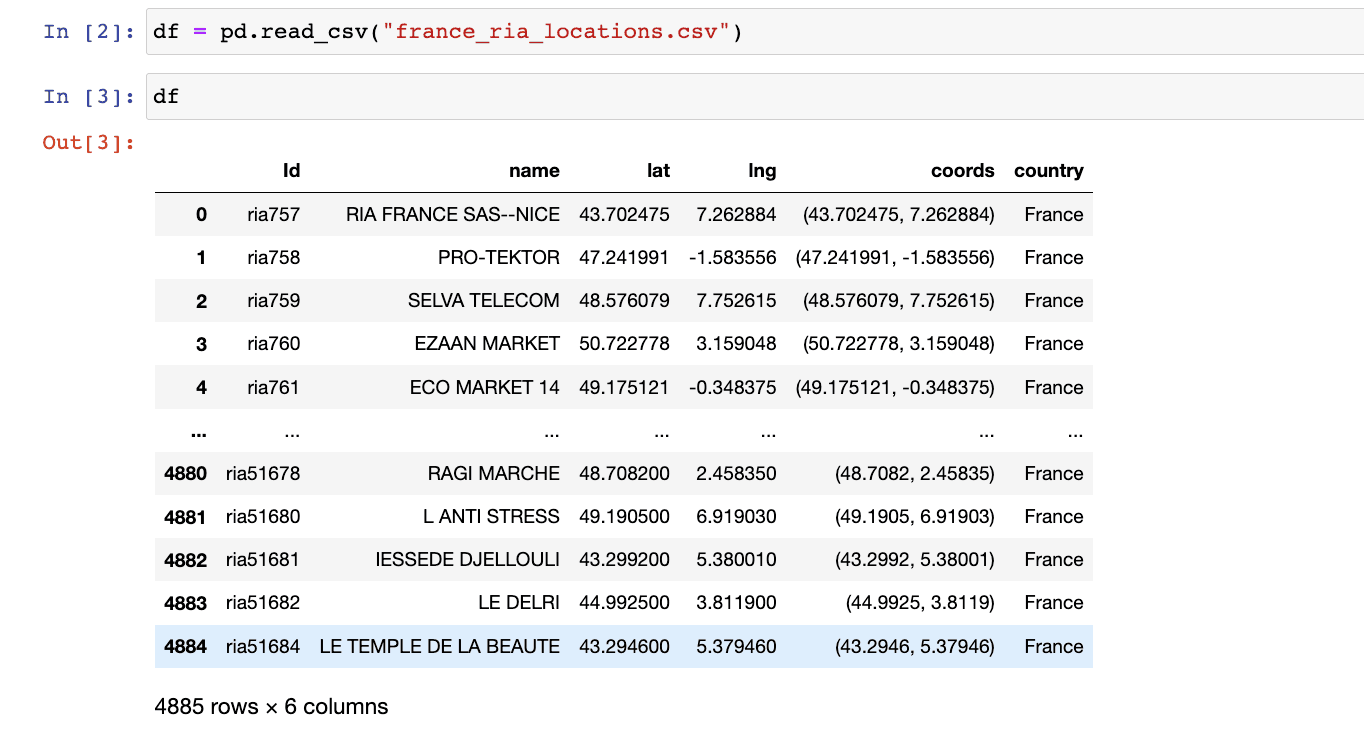
I imported csv file with geo coordinates to pandas DataFrame and I already had coords pair as a string. So, we will need to convert it to a tuple of floats, but first let's initialize Nominatim geocoder.
# Initialize the geocoder
geolocator = Nominatim(user_agent="myGeocoder")
and create a rate limiter
# Create a rate limiter
geocode = RateLimiter(geolocator.reverse, min_delay_seconds=1)
CONVERT COORDS TO TUPLE OF FLOATS
## convert 'coords' from string to a tuple of floats
df['coords'] = df['coords'].apply(lambda x: tuple(map(float, x.strip('()').split(','))))
The next step is to add 'location' column to DataFrame by applying Nominatim geocoder to 'coords' column.
# Add 'location' column to dataframe by applying geocode to 'coords' column
df['location'] = df['coords'].apply(geocode)
SHAPING THE DATAFRAME
# Add 'address', 'city' and 'zip' columns
df['address'] = df['location'].apply(lambda loc: loc.raw['address']['road'] if 'road' in loc.raw['address'] else None)
df['city'] = df['location'].apply(lambda loc: loc.raw['address']['town'] if 'town' in loc.raw['address'] else None)
df['zip'] = df['location'].apply(lambda loc: loc.raw['address']['postcode'] if 'postcode' in loc.raw['address'] else None)
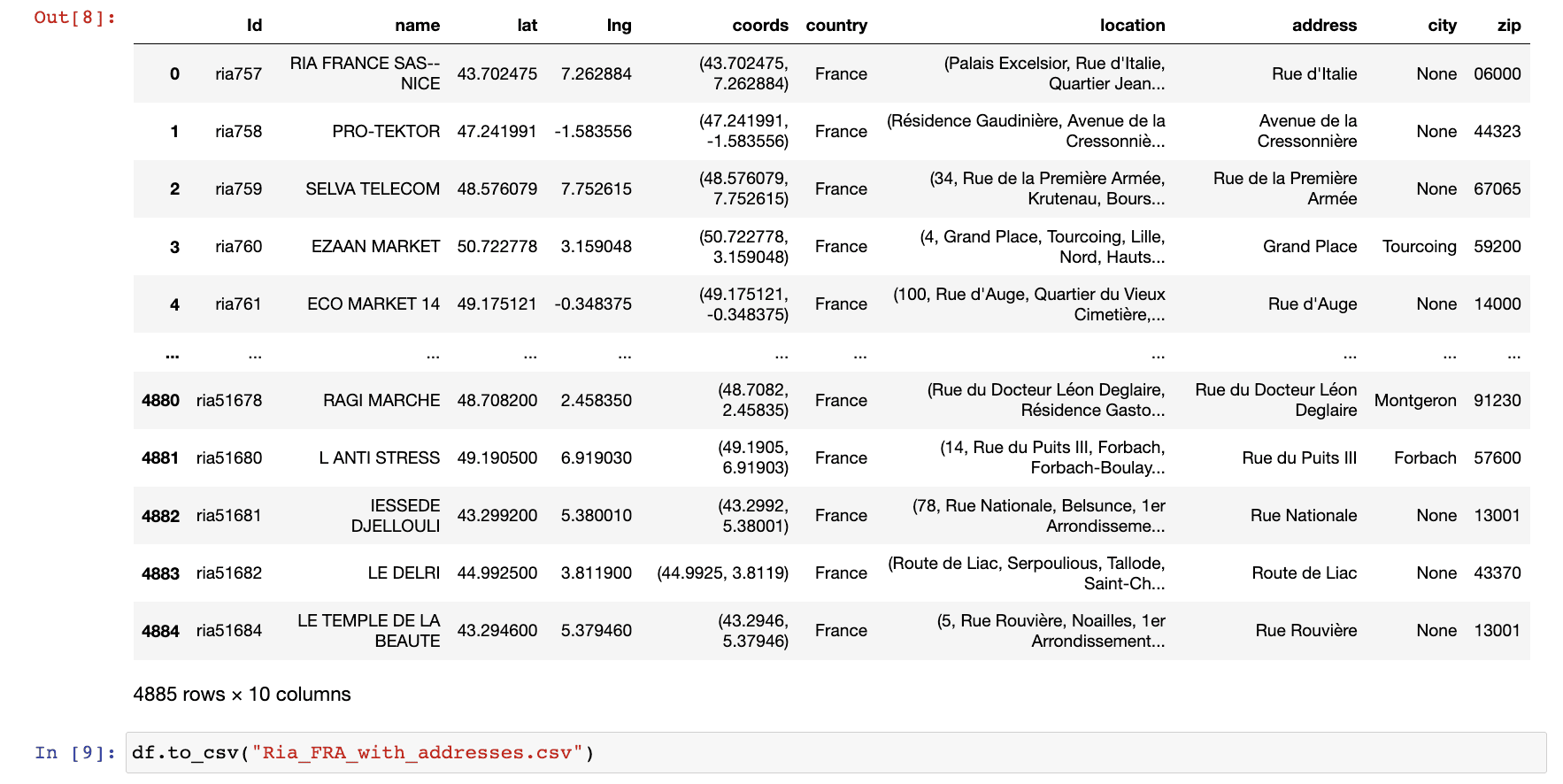
SAVE TO CSV
# Save to csv
df.to_csv('france_ria_locations_with_address.csv', index=False)
Happy coding!